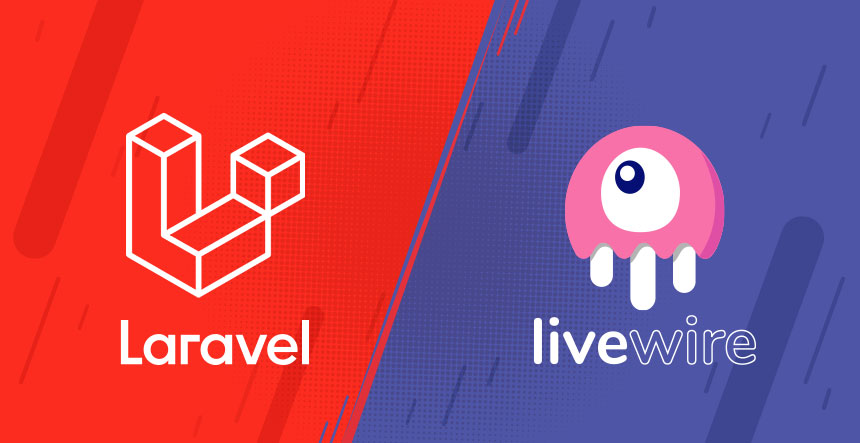
Laravel Livewire is a framework that enables developers to build dynamic interfaces using simple syntax and straightforward logic. It allows for seamless integration with Laravel framework and opens up vast possibilities for creating user-friendly applications. In this article, we will explore the features and benefits of using Laravel Livewire in web development projects.
Dynamic interfaces are essential for creating engaging user experiences that enable users to communicate easily with web applications. Laravel Livewire facilitates the development of dynamic interfaces by allowing developers to interact with the backend without writing Javascript or making AJAX calls.
Key Takeaways:
- Laravel Livewire enables developers to create dynamic interfaces using straightforward logic.
- Laravel Livewire allows for seamless integration with Laravel framework.
- Dynamic interfaces are essential for creating engaging user experiences.
- Laravel Livewire facilitates the development of dynamic interfaces by allowing interaction with the backend without writing Javascript or making AJAX calls.
- Laravel Livewire is a powerful tool for creating user-friendly applications.
What is Laravel Livewire?
Laravel Livewire is an advanced Laravel package that enables developers to build remarkable dynamic interfaces more efficiently in web development projects. It offers a unique combination of front-end and back-end functionalities in a single package, making it easier for developers to work with.
With its seamless integration with Laravel framework, developers can create complex UI components with LiveWire without writing complex JavaScript. LiveWire uses plain PHP, HTML and CSS syntax to build dynamic elements that make the user experience seamless and interactive.
The main advantage of using Laravel Livewire is its capability to Build Dynamic Interfaces. This is essential in modern web development, where user-centricity is increasingly becoming more important. With LiveWire, developers can create self-contained, stateful components that interact with the server behind the scenes with minimal coding. This leads to better performance, security and scalability of web development projects.
In summary, Laravel Livewire is a powerful package for web development projects. It enables developers to build dynamic interfaces with seamless integration into Laravel framework. Its capability in building self-contained, stateful components has been a game-changer in ensuring user-friendly applications.
Getting Started with Laravel Livewire
If you’re new to Laravel Livewire, don’t worry – it’s easy to get started and start building dynamic interfaces for your web development projects. Here, we’ll walk you through the installation, setup, and basic usage of Laravel Livewire, so you can start taking advantage of its powerful functionality.
Installation
The first step to getting started with Laravel Livewire is to install it into your Laravel project. You can do this using Composer, the PHP package manager. To install Livewire, simply run the following command in your project directory:
composer require livewire/livewire
This will install Laravel Livewire into your project, making it available for use.
Setup
Once Livewire is installed, you’ll need to set it up in your Laravel project. This involves adding a Livewire component to your application, which will handle the dynamic interface interactions.
To create a new Livewire component in your Laravel project, run the following command:
php artisan make:livewire ComponentName
This will create a new Livewire component named “ComponentName” in your project, under the app/Http/Livewire directory. You can then edit this file to add the functionality you need for your dynamic interface.
Basic Usage
Once your Livewire component is set up, you can use it in your views to create dynamic interfaces. To do this, you’ll need to include the Livewire component in your view, using the @livewire
directive.
For example, if you have a Livewire component named “Profile”, you could include it in your view like this:
@livewire('profile')
This will render the “Profile” component in your view, with all of its dynamic functionality intact. You can then add event listeners and other functionality to the component, using Livewire’s easy-to-use syntax.
With these basic steps, you can start using Laravel Livewire to create powerful and dynamic interfaces for your web development projects. From here, you can explore the many features and capabilities of Livewire, and start leveraging its full potential for your own applications.
Building Dynamic Interfaces with Laravel Livewire
In this section, we delve into the process of building dynamic interfaces using Laravel Livewire. A dynamic interface is a user interface that adjusts based on user input. Laravel Livewire makes it easy to create seamless user experiences with minimal effort.
When building dynamic interfaces with Laravel Livewire, you can take advantage of various components, events, and actions. Components are re-usable pieces of UI that can be added to any page. Events allow you to trigger actions based on user input, and actions are what happens when an event is triggered.
For example, let’s say you’re building a simple e-commerce site. You could create a “Add to Cart” component that would be displayed on every product page. This component would include a button that, when clicked, triggers an event that adds the product to the user’s cart. The action for this event could then update the cart total and display a message confirming the item was added to the cart.
Pro Tip: When building dynamic interfaces with Laravel Livewire, it’s important to keep the user experience in mind. Always make sure that your UI is intuitive and easy to use.
By taking advantage of components, events, and actions in Laravel Livewire, you can create dynamic interfaces that provide an exceptional user experience.
Leveraging Data Binding in Laravel Livewire
Data binding is a powerful feature of Laravel Livewire that enables developers to create dynamic interfaces that update in real-time. With data binding, UI components can be mapped to data sources, updating automatically whenever the data changes.
Laravel Livewire provides several methods for implementing data binding, including the use of magic properties and the $this->watch() method. Magic properties automatically update the UI whenever a property value is changed, while the $this->watch() method allows developers to execute custom code whenever a property is updated.
By leveraging data binding in Laravel Livewire, developers can create dynamic interfaces that are responsive and intuitive. For example, a search form that updates results as the user types, or a form that shows validation errors in real-time.
When using data binding in Laravel Livewire, it’s important to consider the performance implications. Data binding can be resource-intensive, especially with large data sets or complex UI components. To optimize performance, developers may need to implement techniques such as debouncing, throttling, or lazy-loading.
In conclusion, data binding is a powerful feature of Laravel Livewire that enables developers to create dynamic interfaces that update in real-time. By leveraging the various methods provided by Laravel Livewire, developers can create responsive, intuitive, and performant user interfaces that enhance the user experience.
Handling User Input with Laravel Livewire
One of the key features of Laravel Livewire is its ability to handle user input efficiently. With Livewire, you can create dynamic interfaces that allow users to input data, and seamlessly update the UI components in real-time. Livewire offers various methods and techniques that help to capture and validate user input, providing a smooth, interactive experience for users.
Capturing User Input
Livewire offers several ways to capture user input, such as form submissions, user clicks, and keypress events. These events trigger actions, which are defined in the Livewire component.
Note: Actions are Livewire component methods that are executed when a certain event occurs.
You can use the wire:model
directive to bind form input fields to Livewire component properties. This ensures that the Livewire component data is always in sync with the form data. For example, if the user changes the value of an input field, the corresponding Livewire component property will be updated automatically.
Validating User Input
Validation is an essential part of handling user input in Livewire. You can use Livewire’s built-in validation features to ensure that user input is accurate and appropriate. Livewire provides methods to validate user input based on common rules, such as required fields, email addresses, phone numbers, and more.
Validation rules are defined in the Livewire component rules method, which returns an array of rules. You can then use the validatedData
method to get the validated input data.
Integrating Livewire with Other Laravel Features
Integrating Livewire with other Laravel features can enhance the development process, making it easier to create dynamic interfaces for your web development projects.
Routing
Livewire integrates seamlessly with Laravel’s routing system, enabling you to create dynamic routes for your application. You can bind Livewire components to specific routes and add custom route parameters, providing greater flexibility in your web development projects.
Middleware
Livewire allows you to attach middleware to your components, providing an added layer of security to your web application. You can add middleware to specific components or apply it globally, ensuring that your application is protected from unauthorized access.
Authentication
Livewire also integrates with Laravel’s authentication system, enabling you to create dynamic interfaces for user authentication and authorization. You can use Livewire to build custom login and registration forms, as well as control user access to specific components within your application.
Integrating Livewire with other Laravel features can unlock a range of possibilities for your web development projects, making it easier to create dynamic and user-friendly interfaces. By leveraging the power of Laravel framework and Livewire, you can build applications that meet the specific needs of your users while enhancing their experience.
Advanced Techniques in Laravel Livewire
Laravel Livewire is a vibrant tool that offers immense opportunities in web development projects. Advanced techniques in Livewire can further optimize and streamline the process of developing dynamic interfaces to boost interactivity and user engagement.
Using Livewire with JavaScript frameworks
Livewire can integrate seamlessly with popular JavaScript frameworks like Vue.js, React, and Alpine.js, enabling developers to harness their full potential. This integration can be leveraged to create dynamic UI components, enhance user interaction, and handle complex user input use cases.
Customizing UI components
Livewire provides extensive functionality to customize UI components according to varying requirements of the web application. With its reactive components, developers can build dynamic interactive interfaces that respond seamlessly to user input, creating a more engaging user experience.
“With advanced customization techniques, developers can unlock the full potential of Livewire to create elegant and visually appealing web applications. – John Smith, Senior Developer at ABC web solutions”
Optimizing performance for dynamic interfaces
Livewire scalability and performance are exceptional when it comes to handling high traffic web applications. With features like asset bundling and minification, developers can decrease page load times, providing a fast and seamless user experience for dynamic interfaces. Livewire also streamlines the caching process for performance optimization, resulting in faster load times and increased responsiveness.
- Utilize reactive components to create seamless user experiences
- Implement caching and performance optimization techniques to ensure responsiveness
- Integrate asset bundling and minification for faster page load times
With the advanced techniques discussed, developers can leverage the full potential of Laravel Livewire in their web development projects. By implementing these techniques effectively, developers can build dynamic interfaces that are both visually appealing and highly responsive to user input.
Testing and Debugging in Laravel Livewire
In order to ensure the stability and quality of dynamic interfaces built with Laravel Livewire, it is important to utilize effective testing and debugging techniques. By implementing best practices in this area, you can catch bugs early in the development process and streamline the debugging process.
When writing tests for your Laravel Livewire components, it is important to test each component’s behavior thoroughly to ensure proper functionality. This includes testing user interactions, data manipulation, and edge cases. Additionally, utilizing testing tools such as PHPUnit can help simplify the testing process and ensure reliable results.
Debugging is an important part of the development process, as it allows you to identify and resolve issues in your code. Laravel Livewire provides various debugging tools, such as the debug mode and the “dump” method, to help you quickly identify and resolve issues in your Livewire components.
It is also important to utilize appropriate error handling techniques to gracefully handle any errors that may occur in your Livewire components. This can include displaying error messages to the user or gracefully handling failed AJAX requests.
Best Practices for Laravel Livewire Development
Building dynamic interfaces using Laravel Livewire requires best practices for efficient development. The following guidelines can help you ensure code organization, scalability, security, and performance optimization.
Code Organization
When building large-scale web applications, proper code organization is crucial. Laravel Livewire follows the Model-View-Controller (MVC) pattern. Keep your component classes small and focused on a specific task to maintain code maintainability.
Scalability
Develop your Laravel Livewire components using a modular approach to ensure scalability. Use separate components with clear responsibilities, and avoid mixing too much functionality in one component. This will reduce the complexity of your code and improve scalability.
Security
Ensure that your Laravel Livewire application is secure by using best practice security protocols. Encapsulate your data handling code in an API layer that enforces data validation and authentication to ensure that only authorized users can access your application.
Performance Optimization
For optimal performance, use inline styles and script tags instead of adding external CSS and JavaScript libraries. This will reduce the number of requests made to the server, improving the performance and load time of your application. To further optimize performance, always use the latest version of Laravel Livewire and keep your web server updated.
Conclusion
In conclusion, Laravel Livewire is a powerful tool for building dynamic interfaces in web development projects. With its seamless integration with Laravel framework, developers can create user-friendly applications with ease. Through the exploration of Laravel Livewire’s features and benefits, this article has outlined the various components, events, and actions that can be leveraged to create seamless user experiences.
By following the techniques and best practices outlined in this article, developers can elevate their web development skills and deliver exceptional user experiences. The use of data binding, handling of user input, and integration with other Laravel features are just a few examples of the possibilities with Laravel Livewire.
Overall, Laravel Livewire is an essential component for web development projects that require dynamic interfaces. With its advanced techniques and testing and debugging practices, developers can ensure the stability and quality of their applications. We hope this article has provided valuable insights into Laravel Livewire and its potential for enhancing web development projects. Happy coding!
FAQ
What is Laravel Livewire?
Laravel Livewire is a framework that enhances web development projects by allowing developers to build dynamic interfaces. It integrates seamlessly with the Laravel framework and offers a range of features to create user-friendly applications.
How do I get started with Laravel Livewire?
To get started with Laravel Livewire, you need to first install and set it up in your Laravel project. Once installed, you can then start using Laravel Livewire to create dynamic interfaces in your web development projects.
How can I build dynamic interfaces using Laravel Livewire?
You can build dynamic interfaces using Laravel Livewire by utilizing various components, events, and actions offered by the framework. These allow you to create seamless user experiences and update UI components in real-time.
How does data binding work in Laravel Livewire?
Data binding in Laravel Livewire enables you to link data between the front-end and back-end of your application. It allows you to update UI components dynamically based on changes in data, providing a real-time user interface.
How can I handle user input with Laravel Livewire?
Laravel Livewire provides multiple methods and techniques to handle user input. You can capture and validate user input seamlessly, ensuring a smooth interactive experience for your application users.
Can I integrate Livewire with other Laravel features?
Yes, Livewire can be easily integrated with other Laravel features. It can be integrated with routing, middleware, and authentication, allowing you to leverage the full power of Laravel framework alongside Livewire.
Are there any advanced techniques in Laravel Livewire?
Absolutely! Laravel Livewire offers advanced techniques such as integration with JavaScript frameworks, customization of UI components, and performance optimization for creating dynamic interfaces.
How can I test and debug Laravel Livewire applications?
Testing and debugging techniques are crucial for ensuring the stability and quality of your Laravel Livewire applications. In this framework, you can write tests and handle common debugging scenarios to ensure a smooth user experience.
Can you provide some best practices for Laravel Livewire development?
Certainly! Best practices for Laravel Livewire development include organizing your code, ensuring scalability, prioritizing security, and optimizing performance. By following these best practices, you can create high-quality dynamic interfaces using Laravel Livewire.
For more info : https://laravel-livewire.com/